Field
Introduction
Field
widget within Supervisely is a type of form which has the ability to contain various other widgets. This feature enables users to organize multiple widgets within a layout. Additionally, users have the option to customize the widget by setting a title
, icon
, title_url
and description
.
Function signature
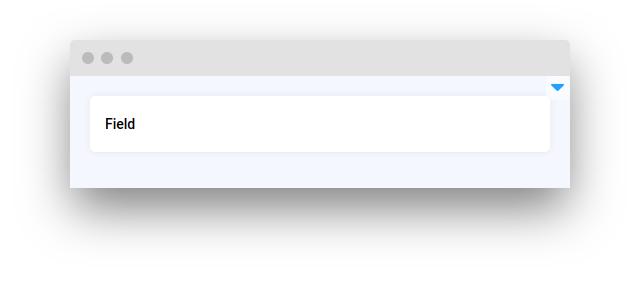
Parameters
Parameters | Type | Description |
---|---|---|
|
| Widget to display on |
|
| Determines |
|
| Determines |
|
| Determines url used on |
|
| Determines url used on |
|
| Determines |
|
| ID of the widget |
content
Determine Widget
to display on Field
.
type: Widget
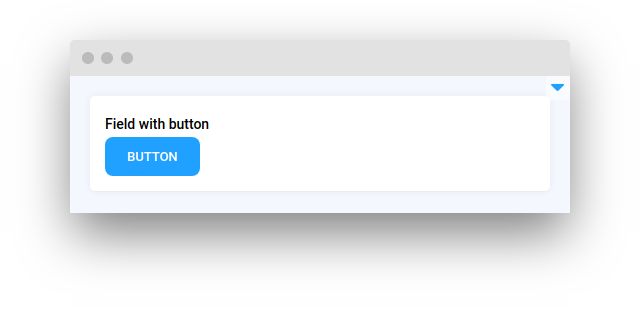
title
Determines Field
title.
type: str
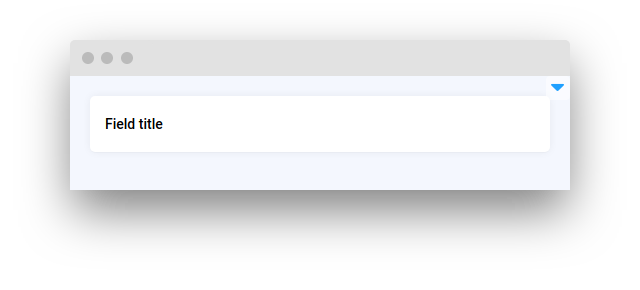
description
Determines Field
description.
type: str
default value: None
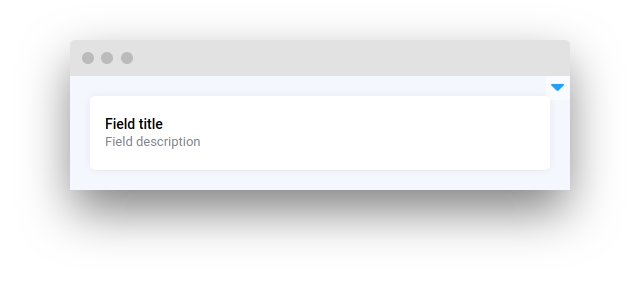
title_url
Determines url used on Field
title.
type: str
default value: None
description_url
Determines url used on Field
description.
type: str
default value: None
icon
Determines Field
icon. Icons can be found at zavoloklom.github.io.
type: Field.Icon
default value: None
widget_id
ID of the widget.
type: str
default value: None
Mini App Example
You can find this example in our Github repository:
ui-widgets-demos/layouts and containers/004_field/src/main.py
Import libraries
Init API client
First, we load environment variables with credentials and init API for communicating with Supervisely Instance:
Initialize Field
widget
Field
widgetCreate app layout
Prepare a layout for app using Card
widget with the content
parameter and place widget that we've just created in the Container
widget.
Create app using layout
Create an app object with layout parameter.
Last updated