Progress
Introduction
This Supervisely widget allows you to display the progress of a task or operation. It is a useful widget for applications that involve long-running processes or tasks that take time to complete.
With the Progress Bar
widget, you can easily create a progress bar that updates in real-time or update the progress bar dynamically as a task progresses, giving users a clear indication of how much time is left until the task is complete.
The Progress Bar Widget can be customized to show progress as percents or values.
Function signature
Progress(
message=None,
show_percents=False,
hide_on_finish=True,
widget_id=None,
)
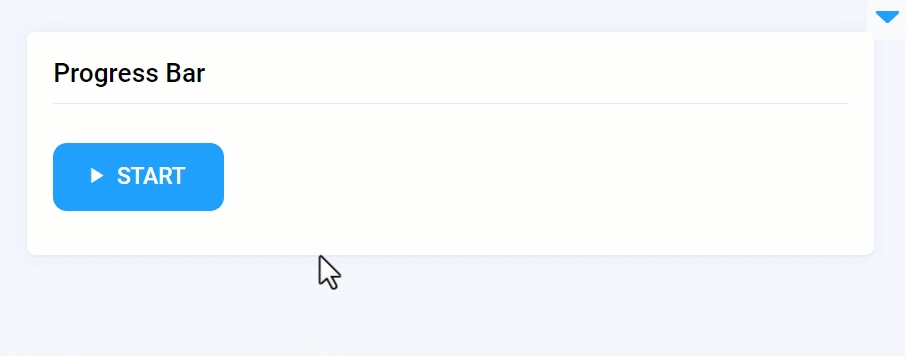
Parameters
message
str
Progress bar message
show_percents
bool
Show progress in percents
hide_on_finish
bool
Hide progress bar on finish
widget_id
str
ID of the widget
message
Progress bar message.
type: str
default value: None
progress = Progress(message="My progress message")
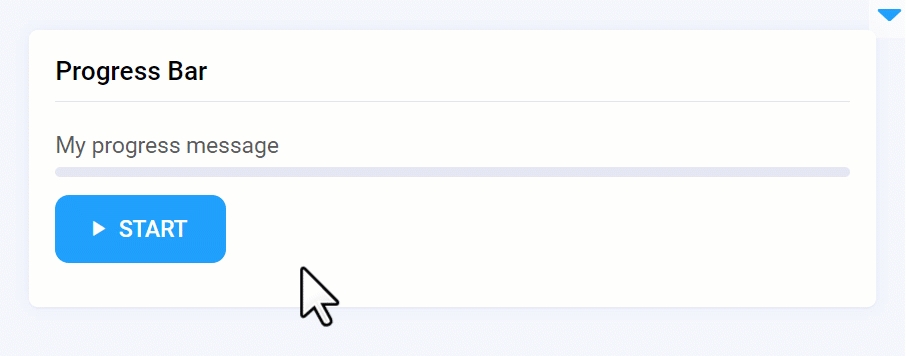
show_percents
Show progress in percents.
type: bool
default value: False
progress = Progress(show_percents=True)
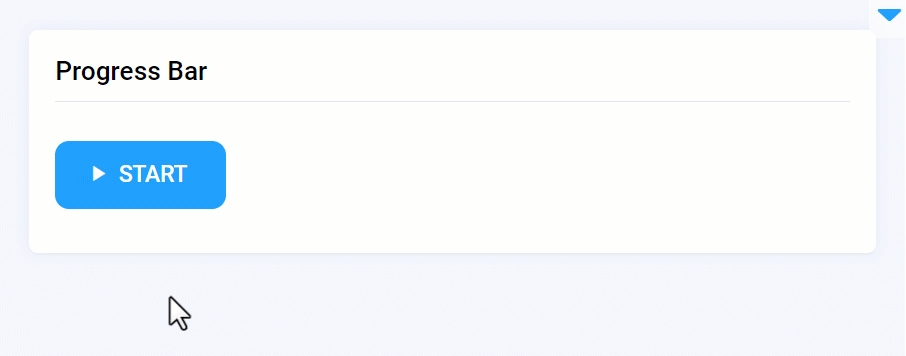
hide_on_finish
Hide progress bar on finish
type: bool
default value: True
progress = Progress(hide_on_finish=True)
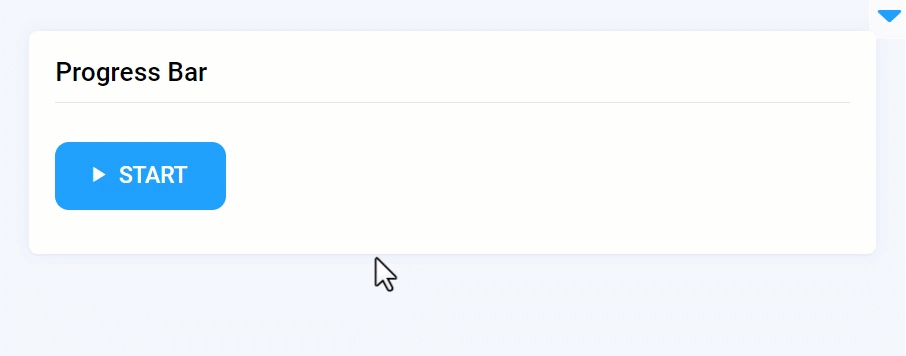
widget_id
ID of the widget.
type: str
default value: None
Mini App Example
You can find this example in our Github repository:
ui-widgets-demos/status elements/001_progress_bar/src/main.py
Import libraries
import os
from time import sleep
import supervisely as sly
from dotenv import load_dotenv
from supervisely.app.widgets import Card, Container
Init API client
Init API for communicating with Supervisely Instance. First, we load environment variables with credentials:
load_dotenv("local.env")
load_dotenv(os.path.expanduser("~/supervisely.env"))
api = sly.Api()
Initialize Progress Bar
, Text
and Button
widgets
Progress Bar
, Text
and Button
widgetsprogress_bar = sly.app.widgets.Progress()
start_btn = sly.app.widgets.Button(text="Start", icon="zmdi zmdi-play")
finish_msg = sly.app.widgets.Text(status="success")
Create app layout
Prepare a layout for app using Card
widget with the content
parameter and place 3 widgets that we've just created in the Container
widget. Place order in the Container
is important, we want the finish message text to be displayed below the progress bar button.
card = Card(
title="Progress Bar",
description="👉 Click on the button to start",
content=Container([progress_bar, start_btn, finish_msg]),
)
layout = Container(widgets=[card], direction="vertical")
Create app using layout
Create an app object with layout parameter.
app = sly.Application(layout=layout)
Our app layout is ready. Progress bar will appear after pressing the Start
button.
Start progress with button click
Use the decorator as shown below to handle button click. Progress Bar
will be updating itself (pbar.update(1)
) every half second by 1 point as specified in sleep
function until it reaches total
10.
@start_btn.click
def start_progress():
with progress_bar(message=f"Processing items...", total=10) as pbar:
for _ in range(10):
sleep(0.5)
pbar.update(1)
finish_msg.text = "Finished"
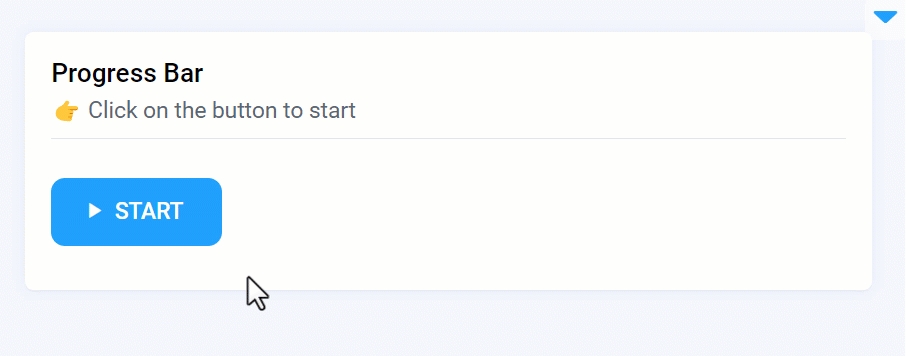
Last updated
Was this helpful?