FileViewer
Introduction
FileViewer
widget in Supervisely is a useful tool for inspecting and easily navigation through files in specific directory in Team files.
Function signature
FileViewer(
files_list=files_list,
selection_type=None,
extended_selection=False,
widget_id=None,
)
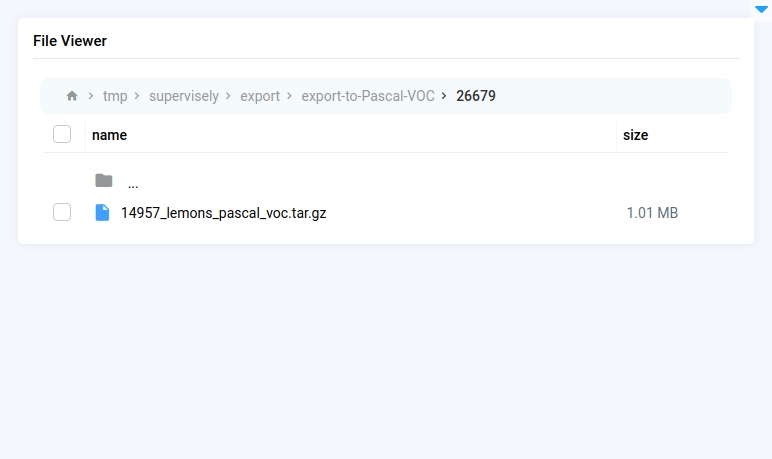
Parameters
files_list
List[dict]
List of dicts with files and folders info
selection_type
Literal[None, "file", "folder"]
Type of data to select
extended_selection
bool
If True
method get_selected_items()
returns a list
of dict
's, instead of list
with paths
widget_id
str
ID of the widget
files_list
Determine pathes to files.
type: List[dict]
files = api.file.list(team_id, path)
tree_items = []
for file in files:
path = file["path"]
tree_items.append({"path": path})
file_viewer = FileViewer(files_list=tree_items)
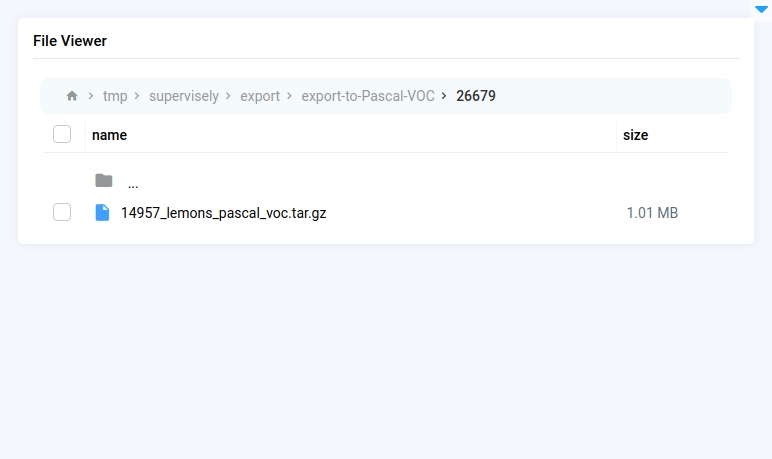
selection_type
Type of data to select. If set to 'file'
- only files can be selected, if set to 'folder'
- only folders can be selected. If set to None
- both files and folders can be selected.
type: Literal[None, "file", "folder"]
default value: None
file_viewer = FileViewer(
files_list=tree_items,
selection_type="file",
)
extended_selection
If True
method get_selected_items()
returns a list
of dict
's, instead of list
with paths.
type: bool
default value: False
file_viewer = FileViewer(
files_list=tree_items,
extended_selection=True,
)
file = file_viewer.get_selected_path()
print(file)
>>> {"path": "some/path", "type": "file or folder"}
widget_id
ID of the widget.
type: str
default value: None
Methods and attributes
loading
Get or set loading
property.
get_selected_items()
Return selected items.
get_current_path()
Return current path to files.
update_file_tree(files_list: List[dict])
Update files tree by given files list.
@path_changed
Decorator function is handled then input path changed.
@value_changed
Decorator function is handled then input value changed.
Mini App Example
You can find this example in our Github repository:
supervisely-ecosystem/ui-widgets-demos/selection/013_file_viewer/src/main.py
Import libraries
import os
import supervisely as sly
from dotenv import load_dotenv
from supervisely.app.widgets import FileViewer, Container, Card, Text
Init API client
First, we load environment variables with credentials and init API for communicating with Supervisely Instance:
load_dotenv("local.env")
load_dotenv(os.path.expanduser("~/supervisely.env"))
api = sly.Api()
Get Team ID from environment variables
team_id = sly.env.team_id()
Prepare file pathes
path = "/" # root of Team Files or specify your directory
files = api.file.list(team_id, path)
tree_items = []
for file in files:
path = file["path"]
tree_items.append({"path": path, "size": file["meta"]["size"]})
Initialize FileViewer
widget
FileViewer
widgetfile_viewer = FileViewer(files_list=tree_items)
Create Text
widgets we will use
Text
widgets we will useselected_values = Text()
curr_path = Text()
Create app layout
Prepare a layout for app using Card
widget with the content
parameter and place widget that we've just created in the Container
widget.
layout = Card(
content=Container(widgets=[curr_path, selected_values, file_viewer]),
title="File Viewer",
)
Create app using layout
Create an app object with layout parameter.
app = sly.Application(layout=layout)
Add function to control widgets from python code
@file_viewer.path_changed
def refresh_tree(current_path):
curr_path.set(
text=f"Current path: {current_path}",
status="text",
)
@file_viewer.value_changed
def print_selected(selected_items):
selected_values.set(
text=f"Selected items: {', '.join(selected_items)}",
status="text",
)
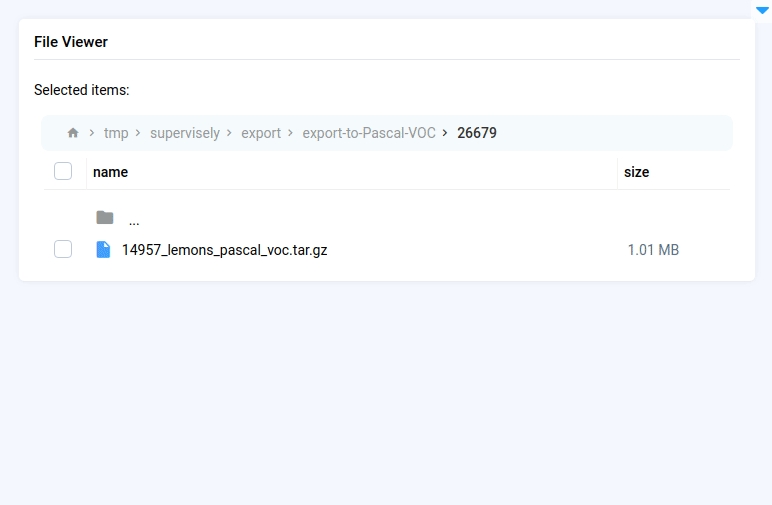
Last updated
Was this helpful?