LabeledImage
Introduction
LabeledImage
widget in Supervisely is designed to display an image with annotations and provides additional features such as the ability to toggle the visibility of individual class annotations, change fill opacity, and includes a zoom function.
Function signature
LabeledImage(
annotations_opacity=0.5,
show_opacity_slider=True,
enable_zoom=False,
esize_on_zoom=False,
fill_rectangle=True,
border_width=3,
widget_id=None
)
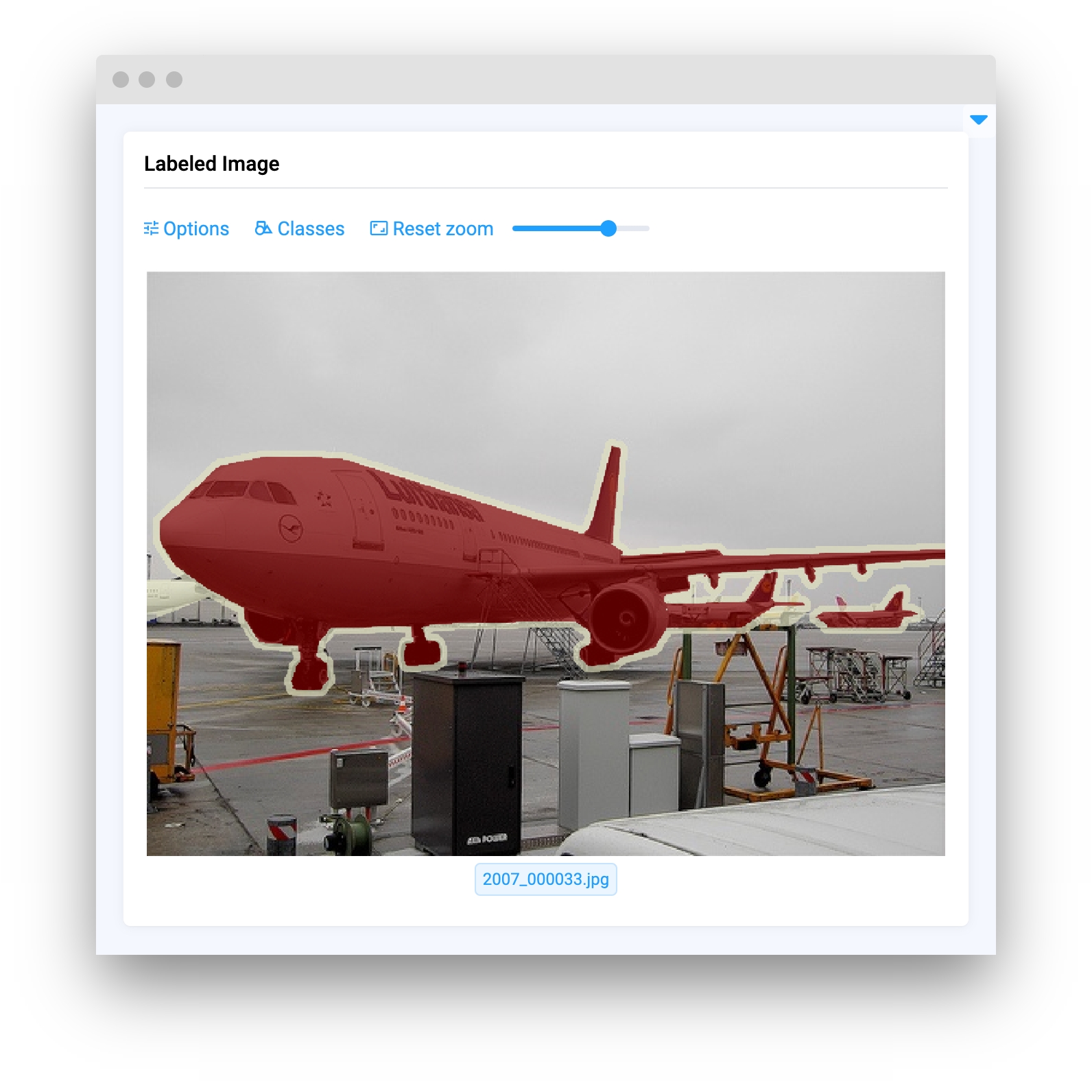
Parameters
annotations_opacity
float
Figures opacity
show_opacity_slider
bool
Determines the presence of opacity slider on LabeledImage
enable_zoom
bool
Enable zoom on LabeledImage
resize_on_zoom
bool
Resize card to fit figure
fill_rectangle
bool
Fill rectange
border_width
int
Border width (thickness)
empty_message
str
If no images are given, this message will be displayed.
widget_id
str
ID of the widget
annotations_opacity
Figures opacity.
type: float
default value: 0.5
labeled_image = LabeledImage(annotations_opacity=1)
labeled_image.set(title=image.name, image_url=image.preview_url, ann=ann)
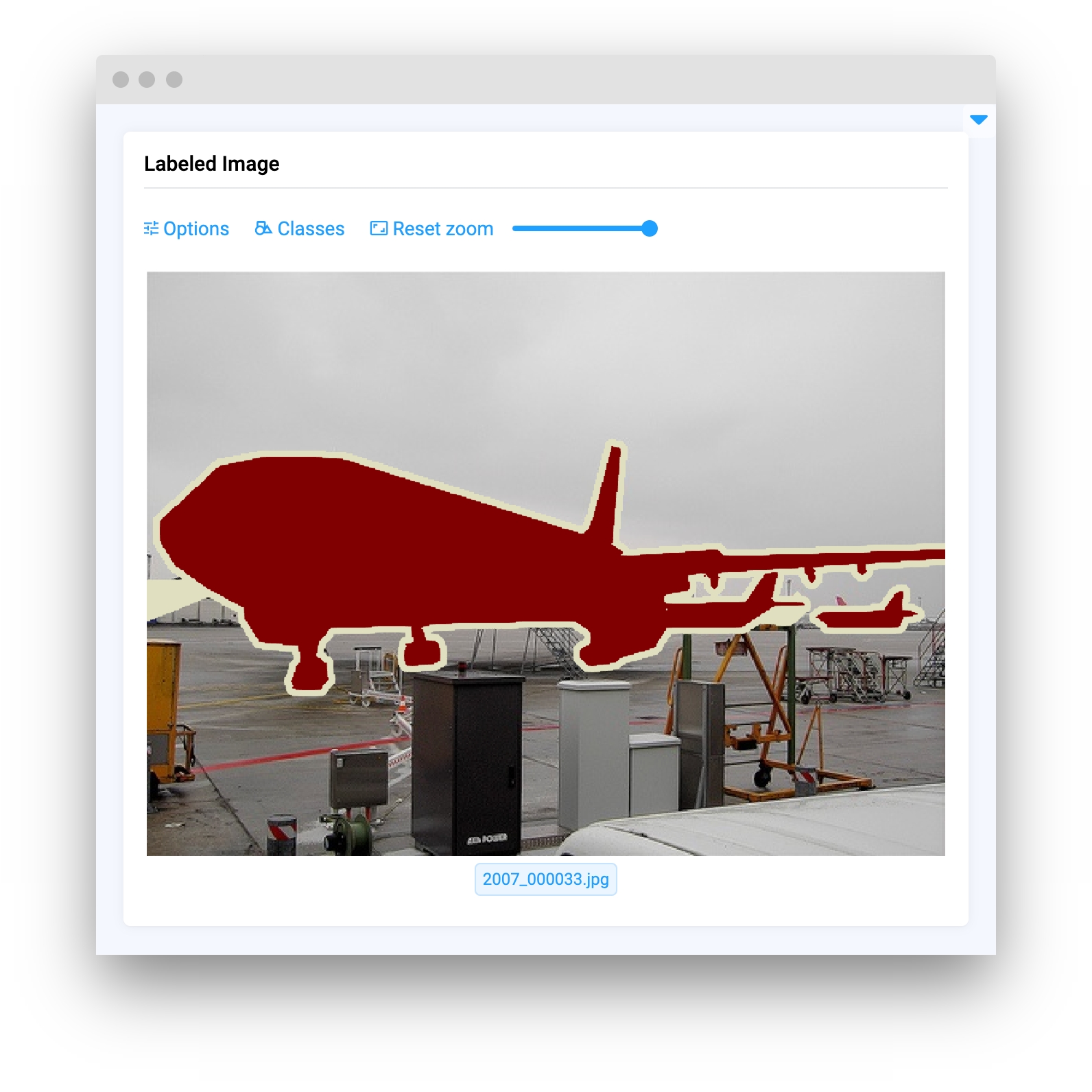
show_opacity_slider
Determines the presence of opacity slider on LabeledImage
.
type: bool
default value: true
labeled_image = LabeledImage(show_opacity_slider=False)
labeled_image.set(title=image.name, image_url=image.preview_url, ann=ann)
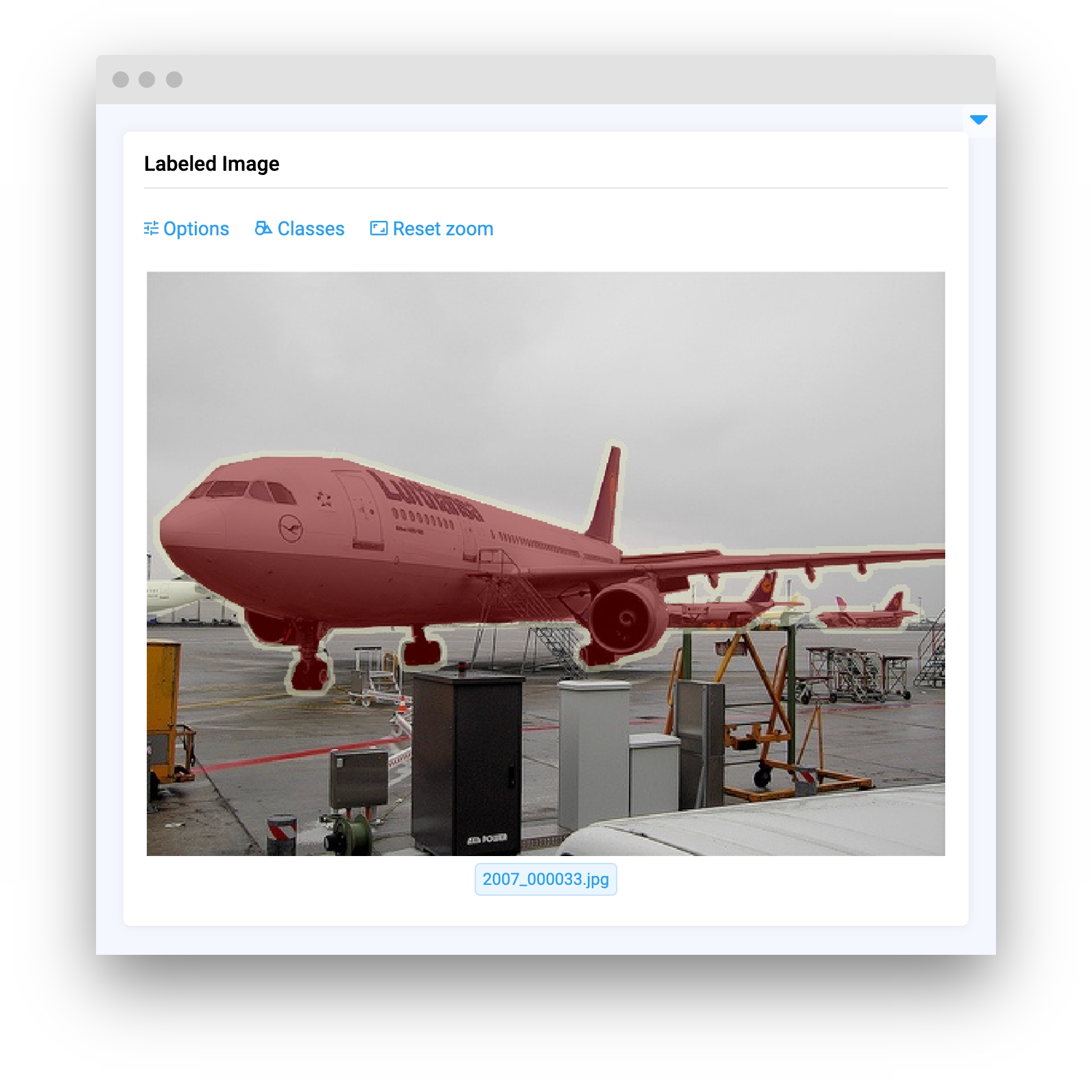
enable_zoom
Enable zoom on LabeledImage
.
type: bool
default value: false
labeled_image = LabeledImage(enable_zoom=True)
labeled_image.set(title=image.name, image_url=image.preview_url, ann=ann)
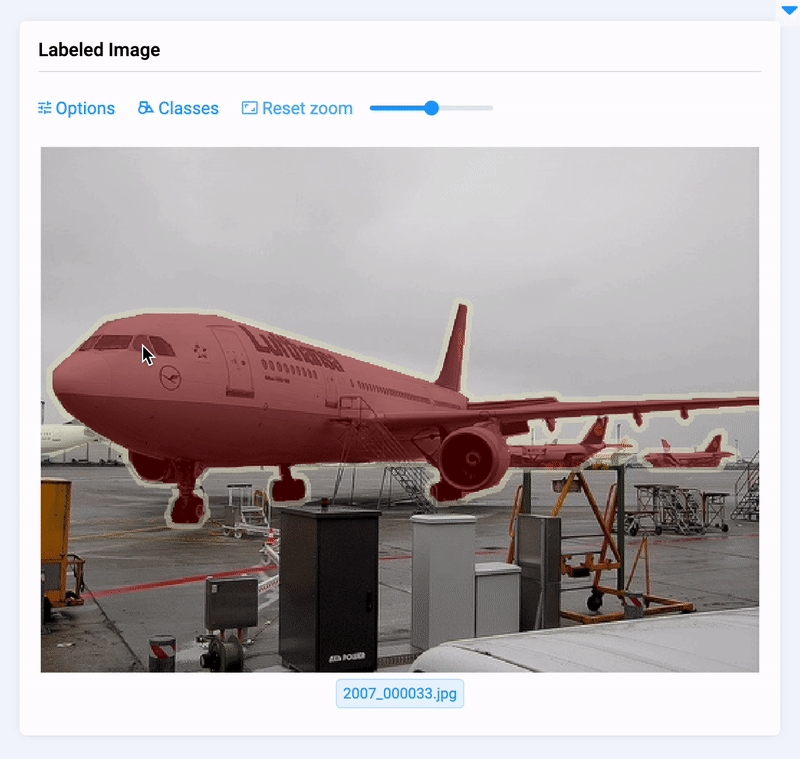
resize_on_zoom
Resize card to fit figure.
type: bool
default value: false
fill_rectangle
Resize card to fit figure.
type: bool
default value: true
labeled_image = LabeledImage()
labeled_image.set(title=image.name, image_url=image.preview_url, ann=ann)
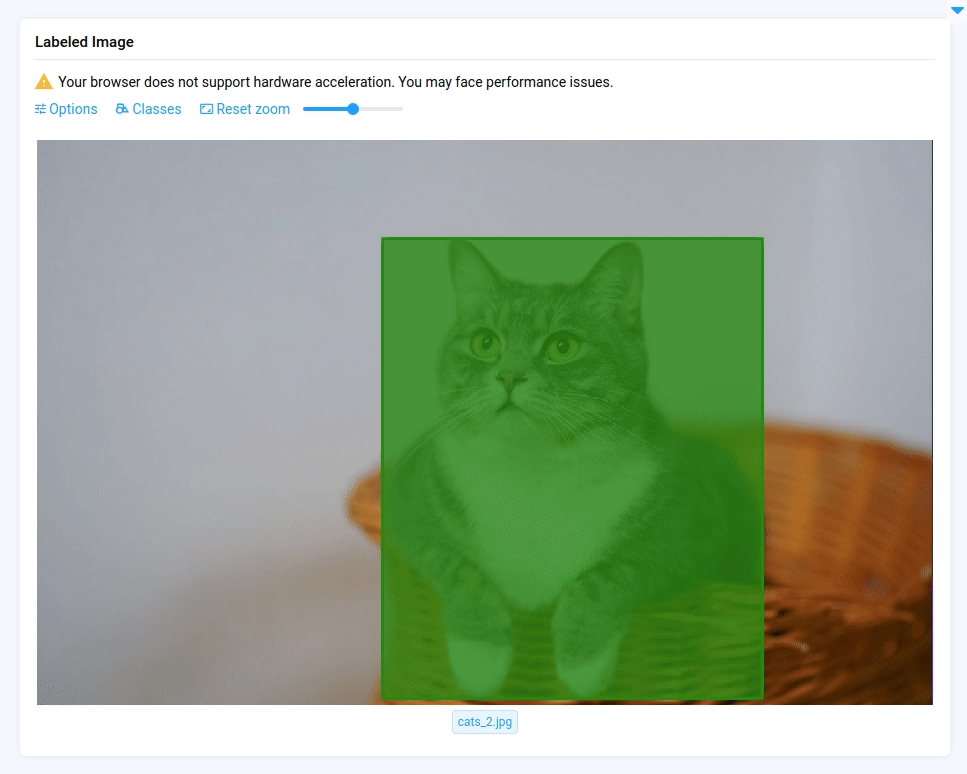
labeled_image = LabeledImage(fill_rectangle=False)
labeled_image.set(title=image.name, image_url=image.preview_url, ann=ann)
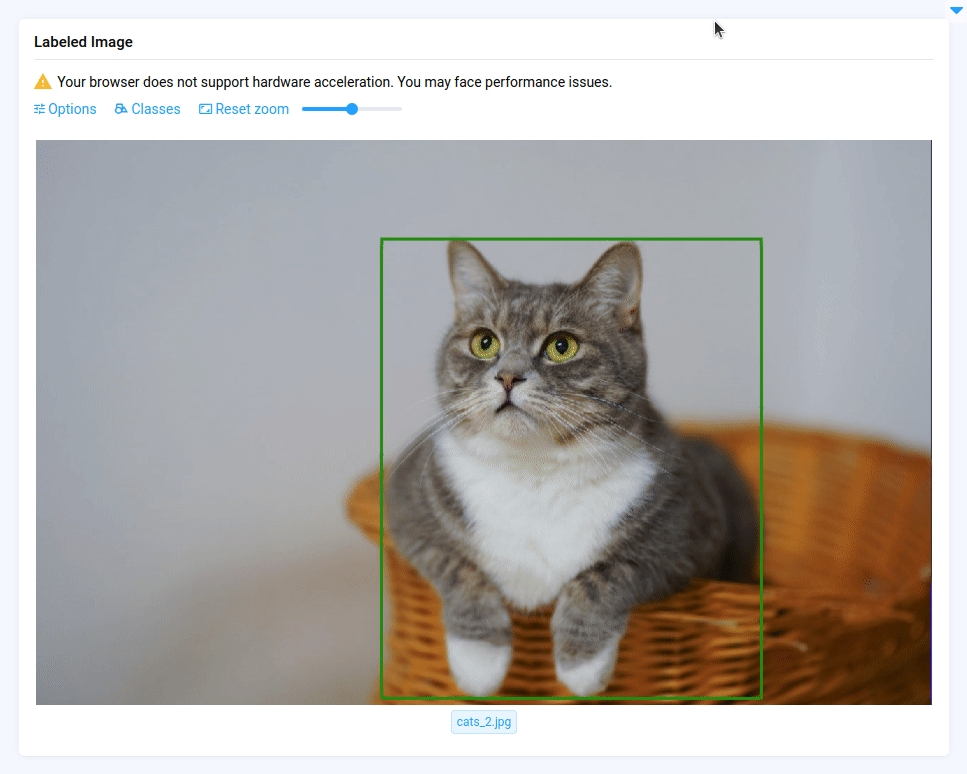
border_width
Resize card to fit figure.
type: int
default value: 3
labeled_image = LabeledImage(border_width=15)
labeled_image.set(title=image.name, image_url=image.preview_url, ann=ann)
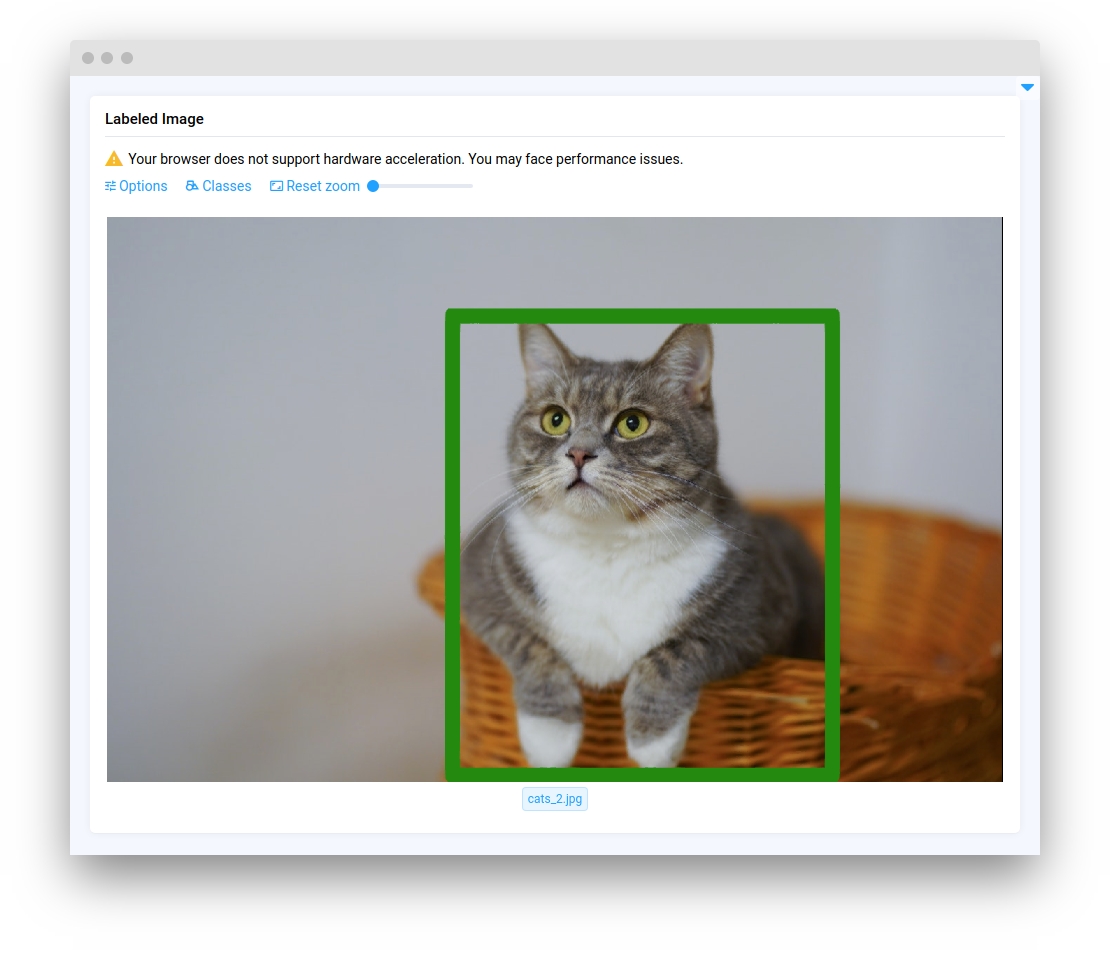
empty_message
If no images are given, this message will be displayed.
type: str
default value: No image was provided
labeled_image = LabeledImage(empty_message="Please provide an image")
widget_id
ID of the widget.
type: str
default value: None
Methods and attributes
id
Get image id property.
loading
Get or set loading
property.
set(title: str, image_url: str, ann: Annotation, image_id: int, zoom_to: int, zoom_factor: float, title_url: str)
Set item in LabeledImage
.
clean_up()
Clean LabeledImage
from item.
is_empty()
Check LabeledImage
is empty.
Mini App Example
You can find this example in our Github repository:
ui-widgets-demos/media/002_labeled_image/src/main.py
Import libraries
import os
import supervisely as sly
from dotenv import load_dotenv
from supervisely.app.widgets import Card, Container, LabeledImage
Init API client
First, we load environment variables with credentials and init API for communicating with Supervisely Instance:
load_dotenv("local.env")
load_dotenv(os.path.expanduser("~/supervisely.env"))
api = sly.Api()
Get project ID and meta
project_id = int(os.environ["modal.state.slyProjectId"])
project = api.project.get_info_by_id(project_id)
meta_json = api.project.get_meta(id=project_id)
meta = sly.ProjectMeta.from_json(data=meta_json)
Get image ID and annotations
image_id = int(os.environ["modal.state.slyImageId"])
image = api.image.get_info_by_id(id=image_id)
ann_json = api.annotation.download_json(image_id=image_id)
ann = sly.Annotation.from_json(data=ann_json, project_meta=meta)
Initialize LabeledImage
widget and fill it with image data
LabeledImage
widget and fill it with image datalabeled_image = LabeledImage()
labeled_image.set(title=image.name, image_url=image.preview_url, ann=ann)
Create app layout
Prepare a layout for app using Card
widget with the content
parameter and place widget that we've just created in the Container
widget.
card = Card(
title="Labeled Image",
content=labeled_image,
)
layout = Container(widgets=[card])
Create app using layout
Create an app object with layout parameter.
app = sly.Application(layout=layout)
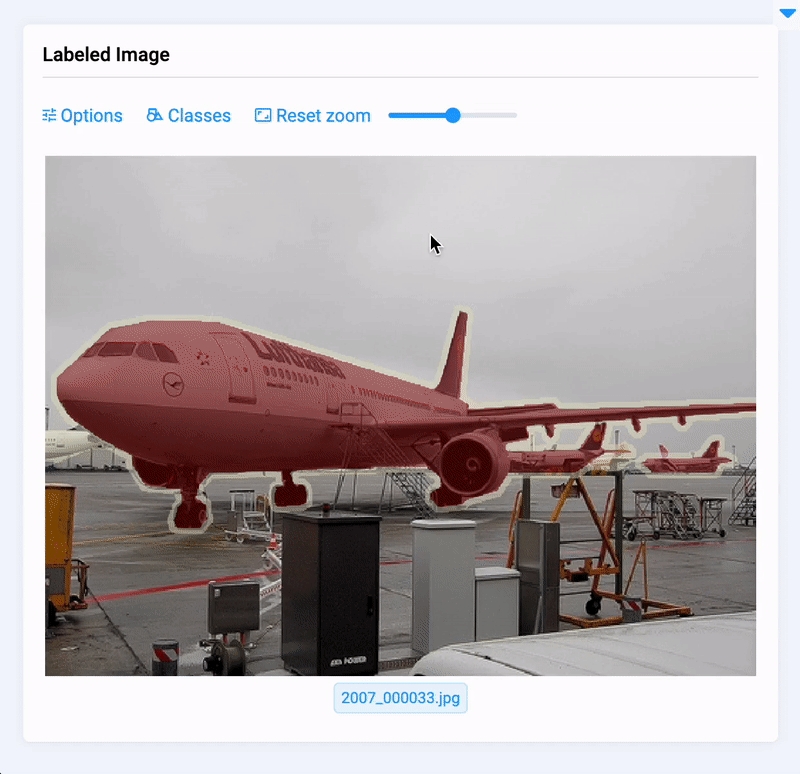
Last updated
Was this helpful?