TeamFilesSelector
Introduction
TeamFilesSelector
is a graphic interface widget in the Supervisely platform that enables users to easily select files and/or folders from their Team files. It allows to customize selecting type (files, folder or both of this types) and displaying additional columns with information about files and folder (size, created date, updated date, type, mime type). The widget features a user-friendly interface and is optimized for performance, making it a valuable tool for teams working.
Function signature
TeamFilesSelector(
team_id=435,
multiple_selection=False,
max_height=500,
selection_file_type=None,
hide_header=True,
hide_empty_table=True,
additional_fields=[],
widget_id=None,
)
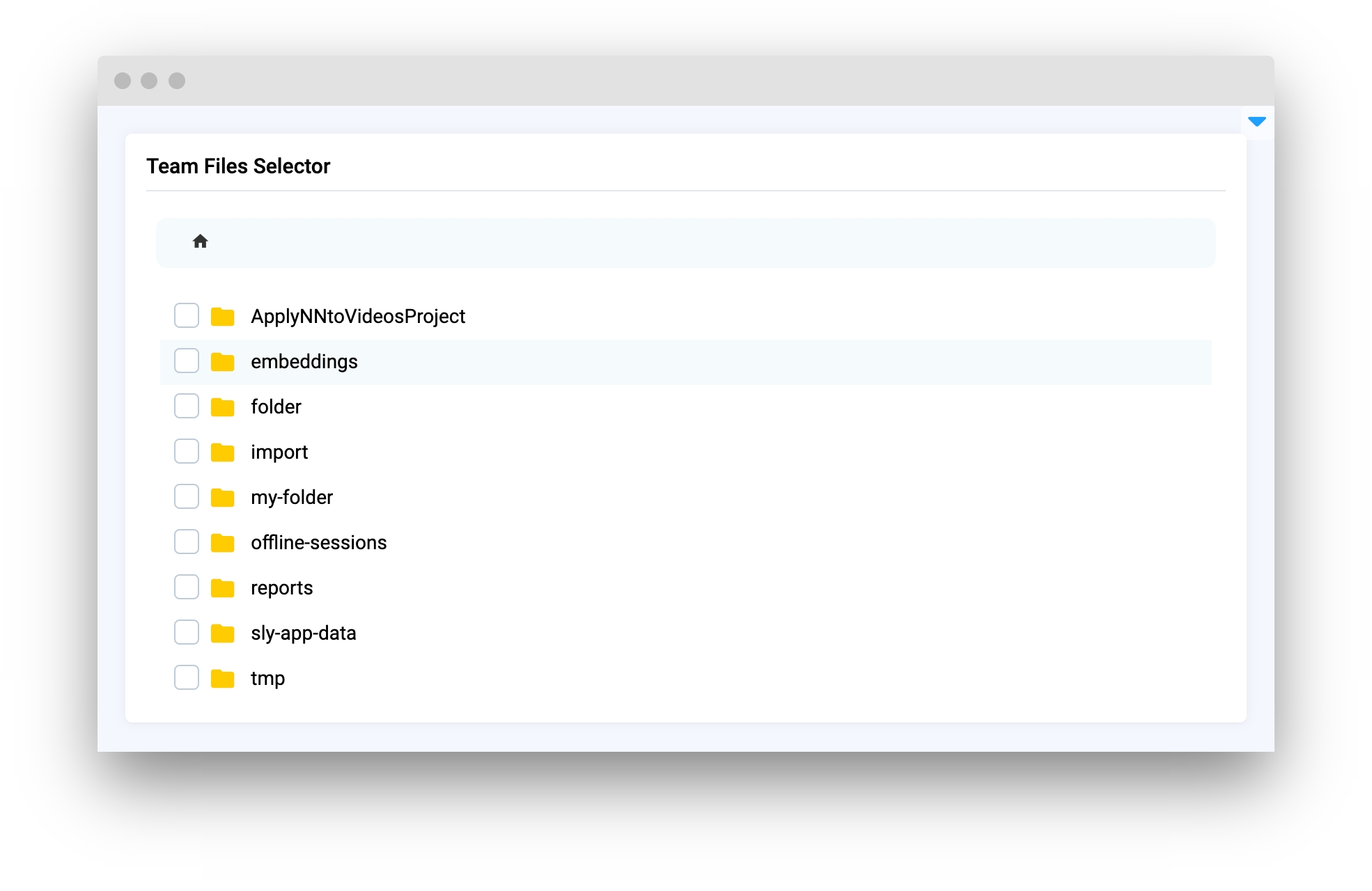
Parameters
team_id
int
Team ID
multiple_selection
bool
Whether available selection multiple files/folders
max_height
int
Determine maximum height of the widget
selection_file_type
Literal["folder", "file"]
or None
Determine type of items available for selection
hide_header
bool
If True
hide widget table header
hide_empty_table
bool
If True
and Team files directory is empty it will display message
additional_fields
List[Literal["id", "createdAt", "updatedAt", "type", "size", "mimeType"]]
Determine column names to display additional information about files/folders
widget_id
str
ID of the widget
team_id
Team ID
type: int
file_selector = TeamFilesSelector(team_id=435)
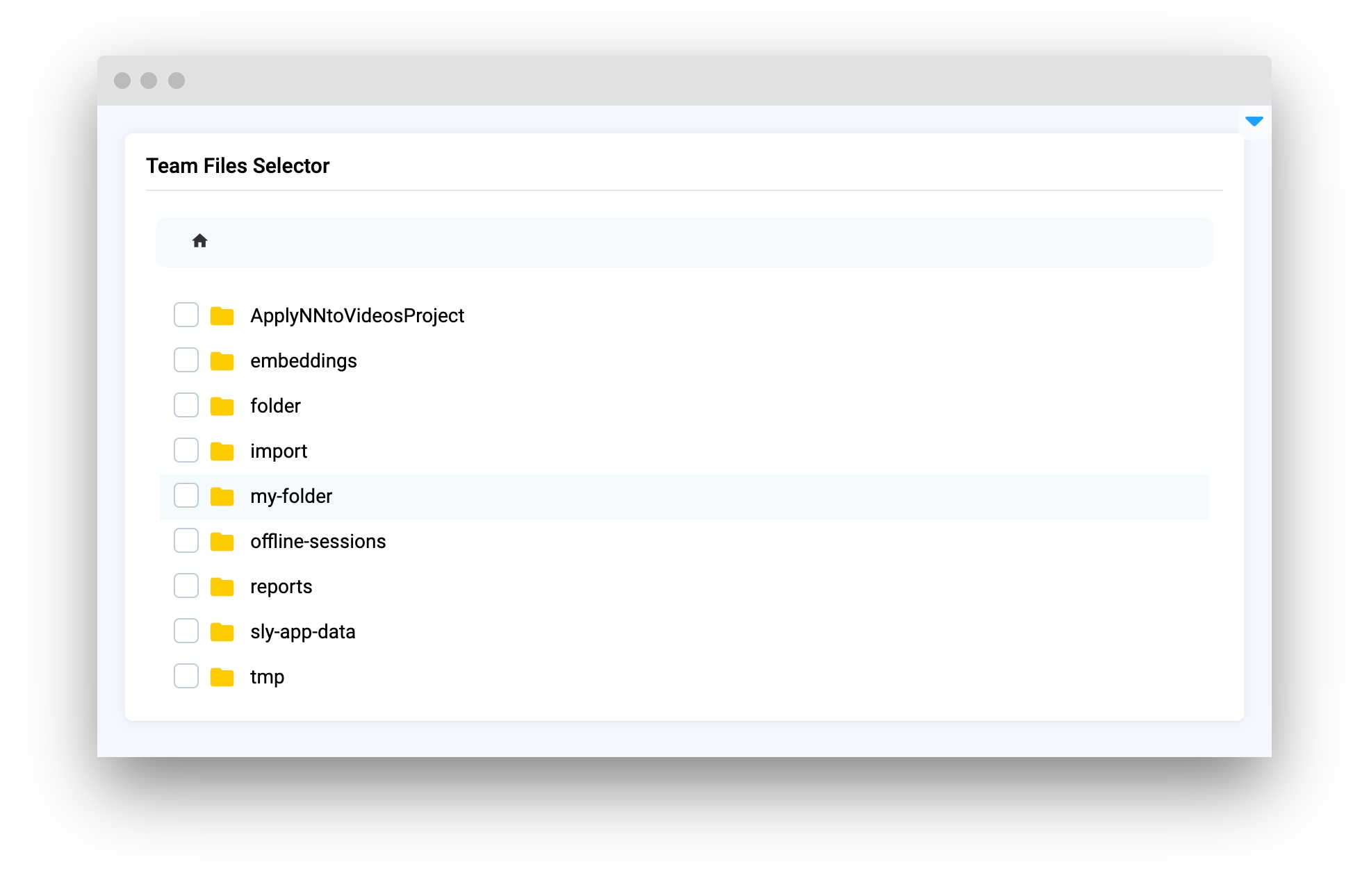
multiple_selection
Whether available selection multiple files/folders
type: bool
default value: False
file_selector = TeamFilesSelector(
team_id=435,
multiple_selection=True,
)
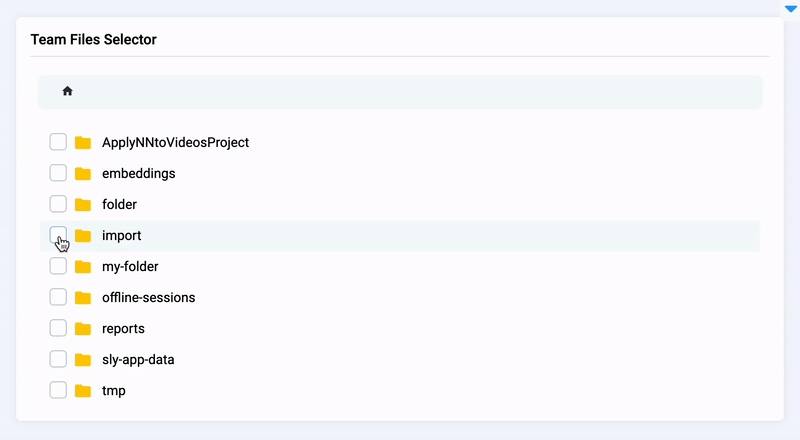
file_selector = TeamFilesSelector(
team_id=435,
multiple_selection=True,
)
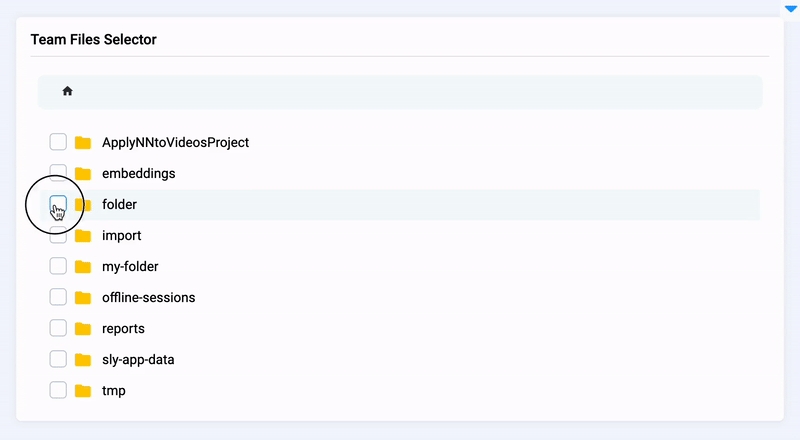
max_height
Determine maximum height of the widget
type: int
default value: 500
file_selector = TeamFilesSelector(
team_id=435,
max_height=200,
)
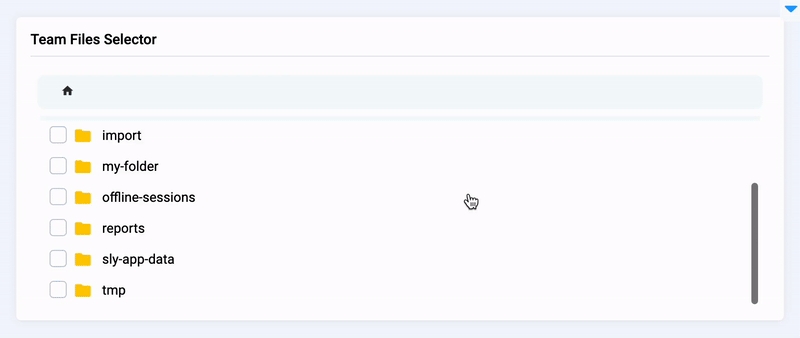
hide_header
If True
hide widget table header
type bool
default value: True
file_selector = TeamFilesSelector(
team_id=435,
hide_header=False
)
selection_file_type
Determine type of items available for selection
type: Literal["folder", "file"]
or None
default value: None
file_selector = TeamFilesSelector(
team_id=435,
selection_file_type=None,
)
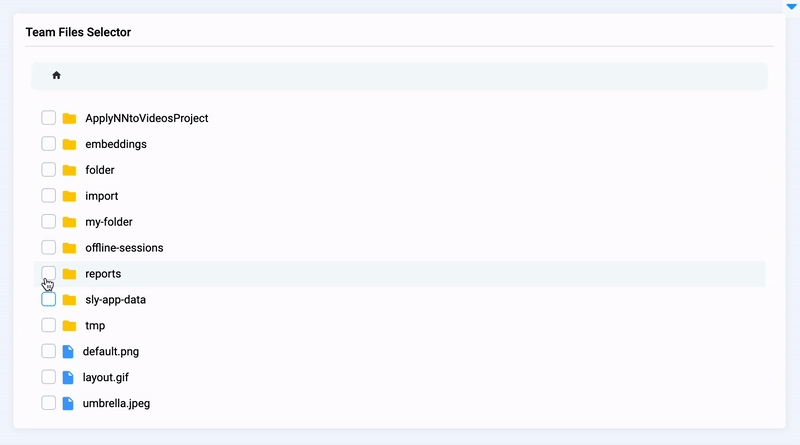
file_selector = TeamFilesSelector(
team_id=435,
selection_file_type="file"
)
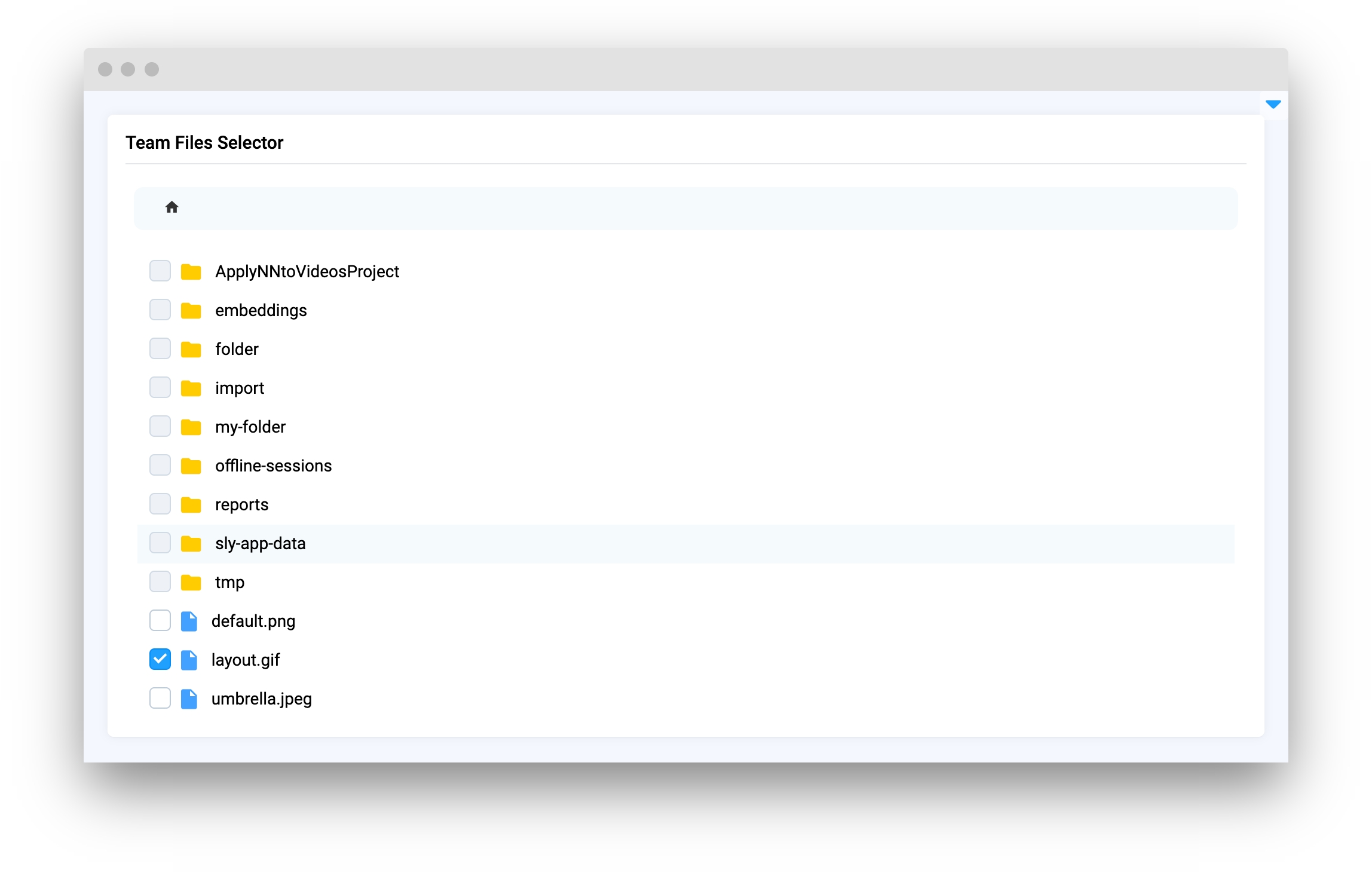
file_selector = TeamFilesSelector(
team_id=435,
selection_file_type="folder"
)
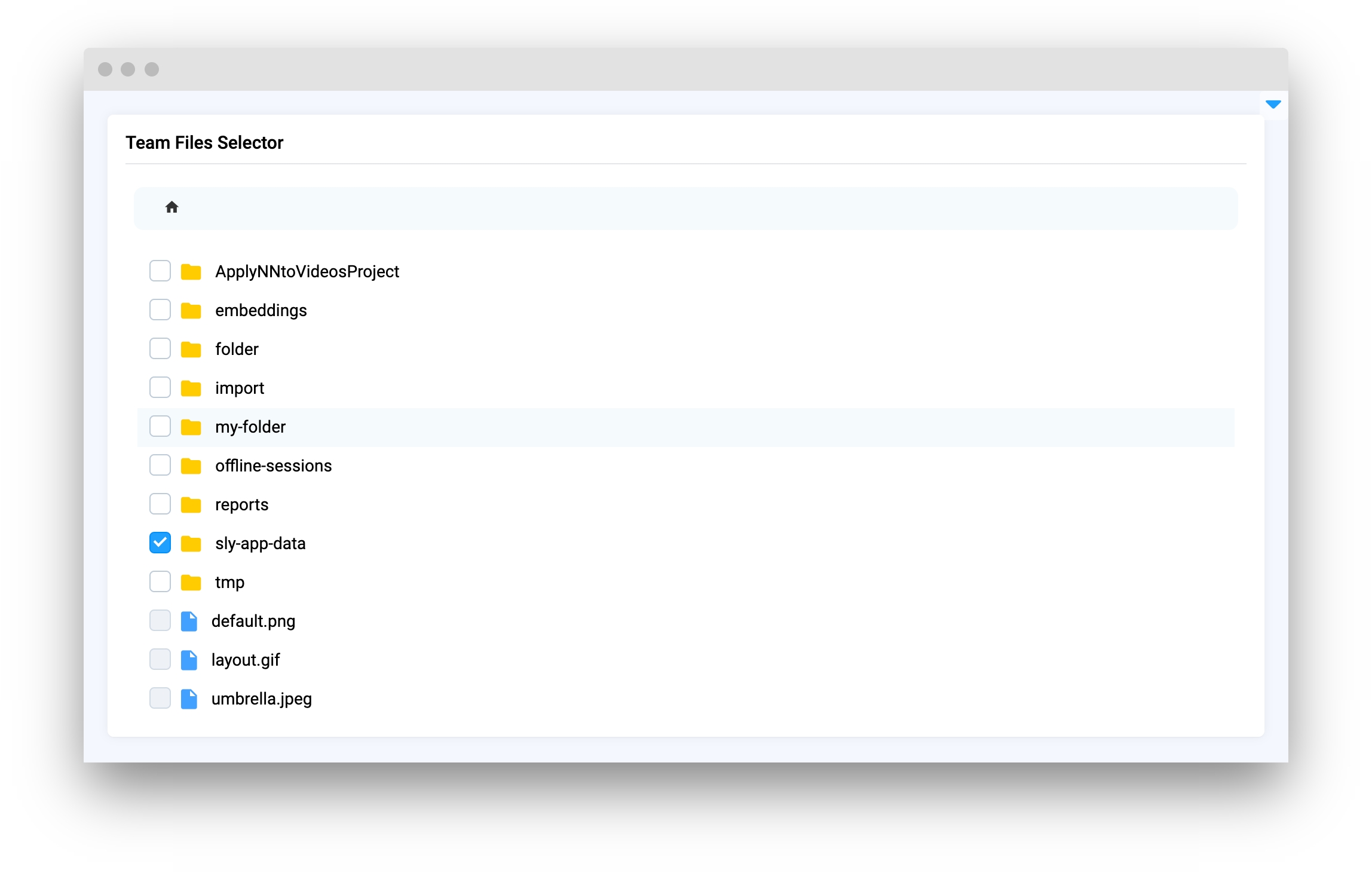
hide_empty_table
If True
and Team files directory is empty it will display message
type: bool
default value: True
file_selector = TeamFilesSelector(
team_id=435,
hide_empty_table=True,
)
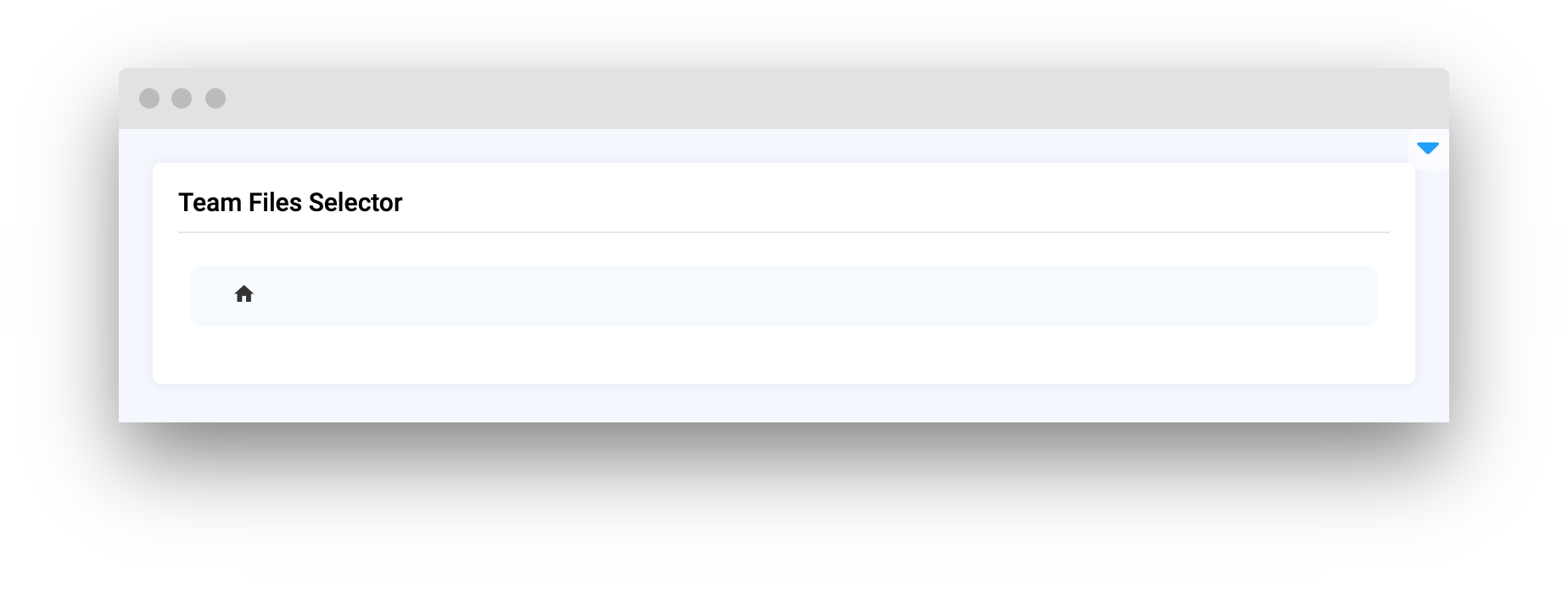
file_selector = TeamFilesSelector(
team_id=435,
hide_empty_table=False,
)
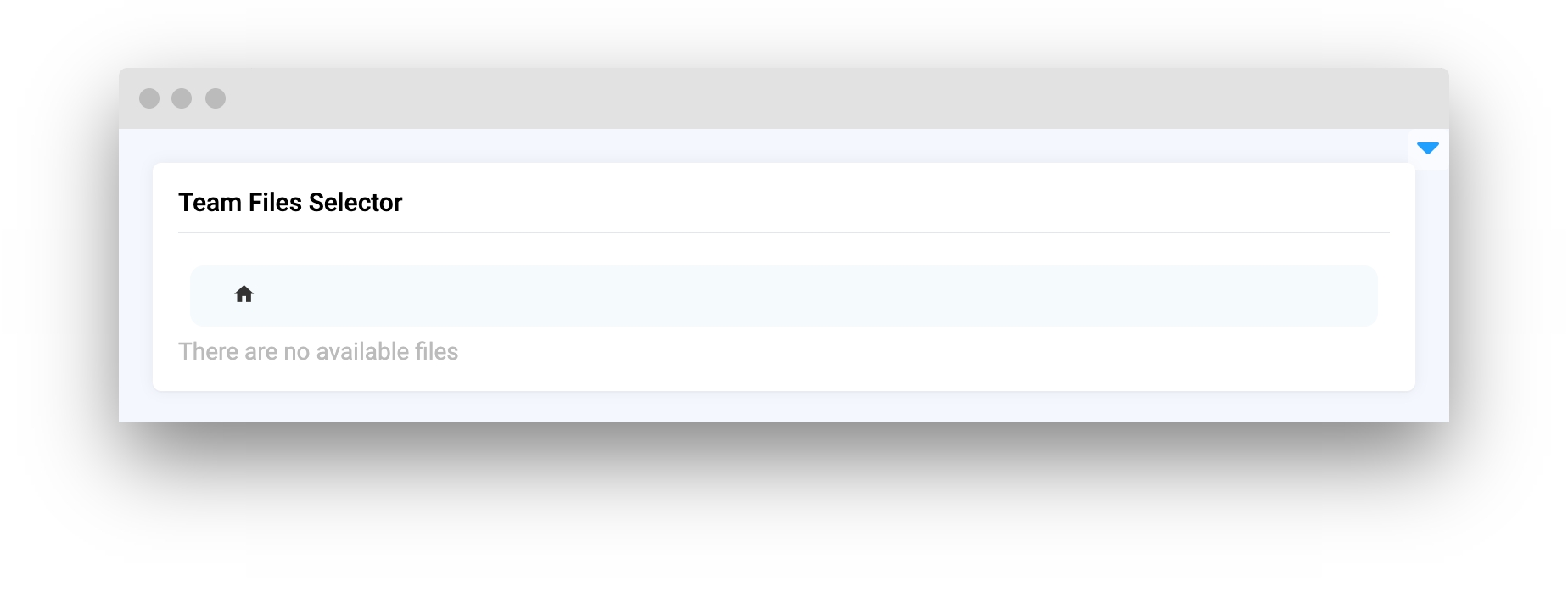
additional_fields
Determine column names to display additional information about files/folders
type: List[Literal["id", "createdAt", "updatedAt", "type", "size", "mimeType"]]
default value: []
file_selector = TeamFilesSelector(
team_id=435,
hide_header=False,
additional_fields=["id", "createdAt", "updatedAt", "type", "size", "mimeType"],
)
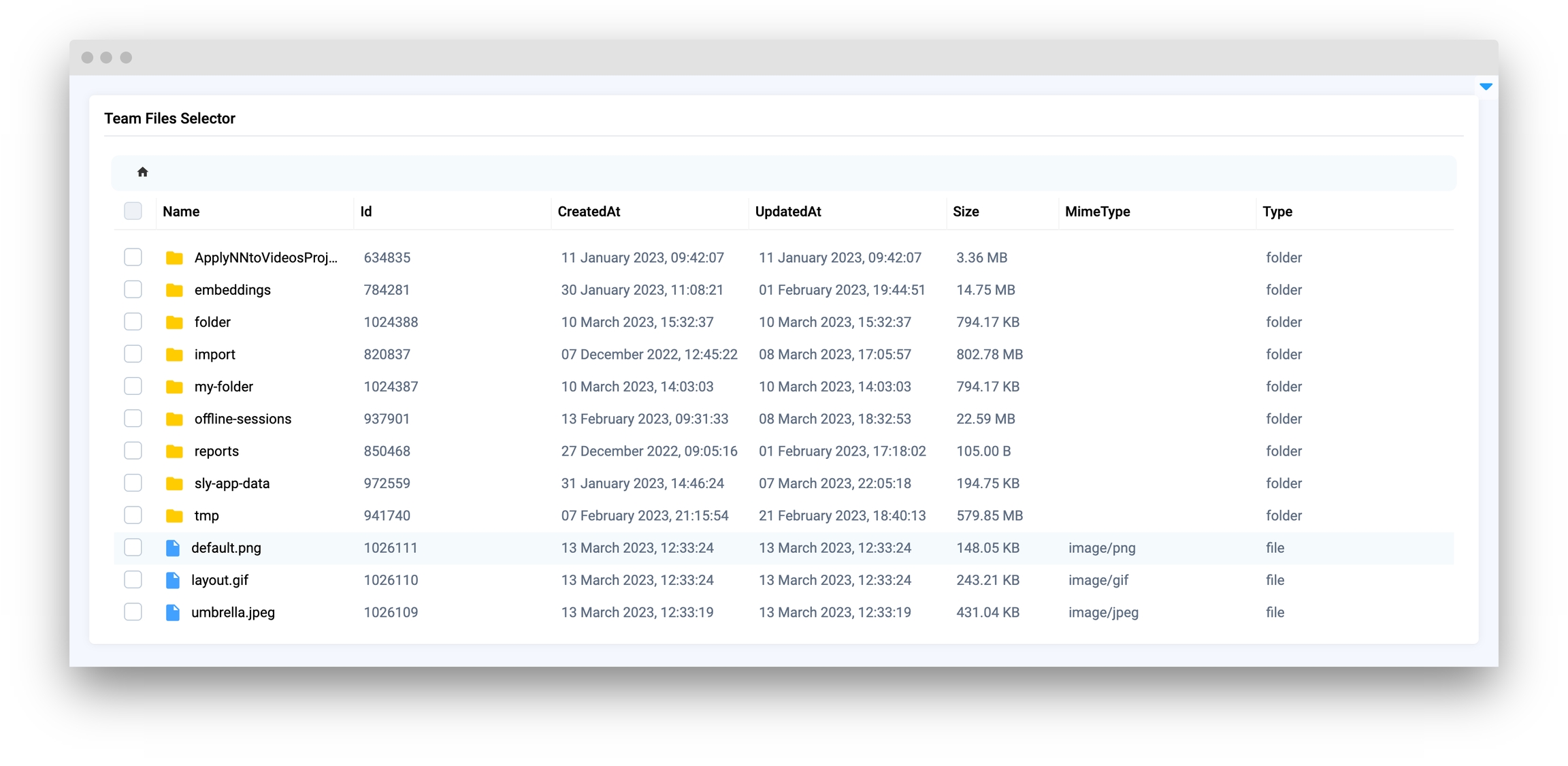
widget_id
ID of the widget
type: str
default value: ``
Methods and attributes
get_selected_paths()
Get list of path for selected files/folders in Team files.
get_selected_items()
Get list of selected files/folders information in Team files.
set_team_id(team_id: int)
Set team_id
for TeamFilesSelector
widget.
Mini App Example
You can find this example in our Github repository:
supervisely-ecosystem/ui-widgets-demos/selection/012_team_files_selector/src/main.py
Import libraries
import os
import supervisely as sly
from dotenv import load_dotenv
from supervisely.app.widgets import Button, Card, Container, TeamFilesSelector, Text
Init API client
First, we load environment variables with credentials and init API for communicating with Supervisely Instance:
load_dotenv("local.env")
load_dotenv(os.path.expanduser("~/supervisely.env"))
api = sly.Api()
Get team_id
from environment variables
team_id
from environment variablesteam_id = sly.env.team_id()
Initialize TeamFilesSelector
widget
TeamFilesSelector
widgetfile_selector = TeamFilesSelector(
team_id=team_id,
max_height=300,
multiple_selection=True,
selection_file_type="folder",
hide_header=False,
additional_fields=["createdAt", "type", "size"],
)
Create Text
, Button
widgets we will use in UI for demo
Text
, Button
widgets we will use in UI for demotext = Text()
button = Button()
Create app layout
Prepare a layout for app using Card
widget with the content
parameter and place widget that we've just created in the Container
widget.
card = Card(
title="Team Files Selector",
content=Container([file_selector, button, text]),
)
layout = Container(widgets=[card])
Create app using layout
Create an app object with layout parameter.
app = sly.Application(layout=layout)
Add functions to control widgets from code
@button.click
def show_selected():
selected_paths = file_selector.get_selected_paths()
text.text = "<br>".join(selected_paths)
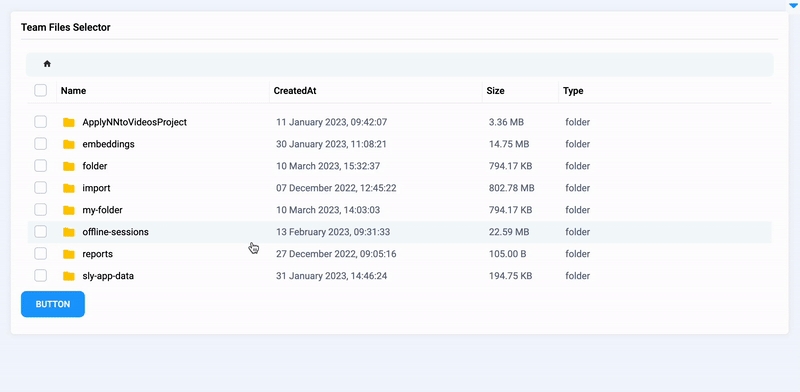
Last updated
Was this helpful?